STL
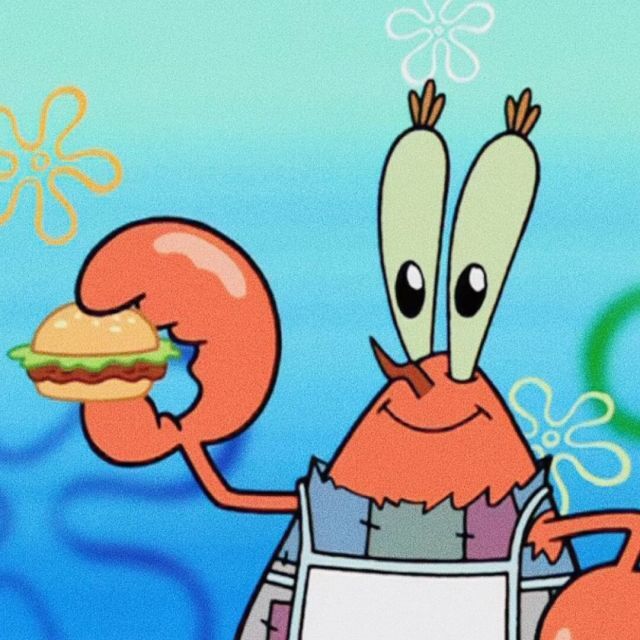
📅time:8.17 - 8.18
🍔题目:
1039 25 Course List for Student
1047 25 Student List for Course very easy
1063 25 Set Similarity set 题目看不懂,做也不会做
1060 30 Are They Equal
1100 20 Mars Numbers
1054 20 The Dominant Color
1071 25 Speech Patterns
1022 30 Digital Library
vector
很多时候题目给的是字符或字符串,需要利用hash或者map进行映射。
使用map可能会超时或内存超限。
set
map
map会以键从小到大排序
需掌握map<string,set<int>>p
判断是否存在某键
mp.count(key==0);
mp.find(key)==mp.end();
删除
mp.erase(it);
迭代器mp.erase(key);
mp.erase(first,last);
迭代器
其它
unordered_map:只映射不按key排序
multimap:一个键可对应多个值(不是很清楚,也许可以用map<typename,set<typename>>
代替)
distinct common numbers
distinct numbers
附录
1039 Course List for Student
注意点:哈希表的使用:将学生名字abc9(由三个字母和一个数字组成)对应到表中。利用map<string,int>可能导致存储过大,提示段错误,因此可考虑hash表的形式。(具体见“需注意的点”map和哈希表)
将学生名字abc9(由三个字母和一个数字组成)对应到表中,利用map<string,int>
可能导致存储过大,提示段错误,因此可考虑hash表的形式。
1 |
|
1047 Student List for Course
1 |
|
1063 Set Similarity
1 |
|
1060 Are They Equal
1100 Mars Numbers
1054 The Dominant Color
1 |
|
1071 Speech Patterns
1022 Digital Library
测试点3、4:注意输出时要”%07d\n”
1 |
|
Comments